Load testing a server
2022 Jun 12
See all posts
Load testing a server
What is load testing? ⁉️
Quoting wikipedia:
Load testing generally refers to the practice of modeling the expected usage of a software program by simulating multiple users accessing the program concurrently.
Let's get started then! 🏁
Requirements
We are gonna load test our server using Locust, a simple and straight forward python library.
Install locust by running:
Test the installation:
Load testing
Create a python file locustfile.py
and add the following code in it:
from locust import HttpUser, task
class HelloWorldUser(HttpUser):
@task
def hello_world(self):
self.client.get("/") #URL that we will request
In the same folder, start testing by running:
You should see something similar to:

Now, you can access http://localhost:8089
You will now see Locust UI
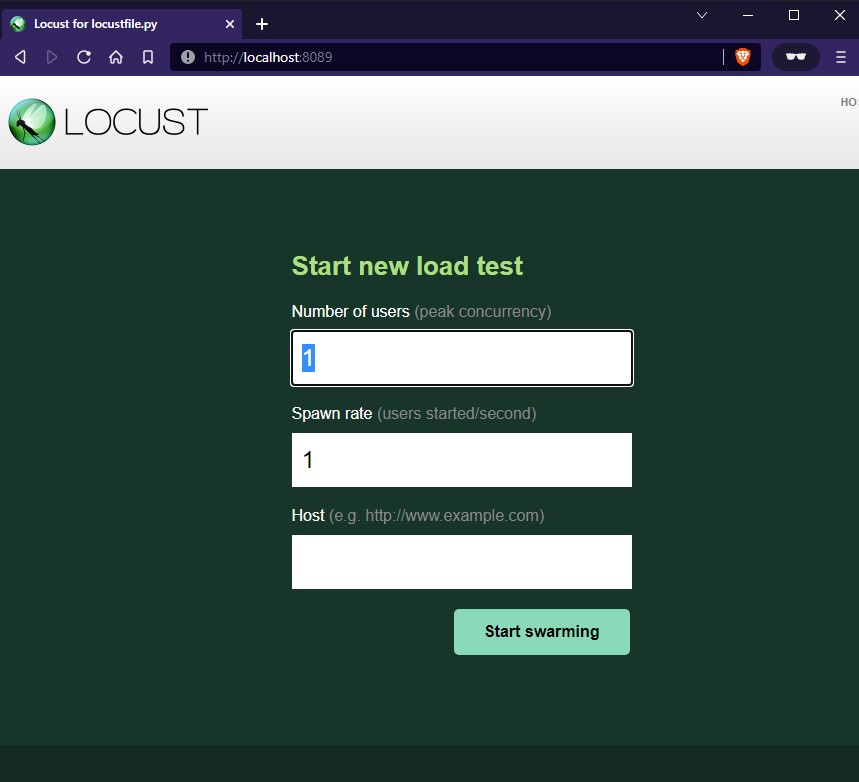
Configure according your preferred settings and off you go! 🏎️
Testing
You will be able to see the stats of your ongoing test:
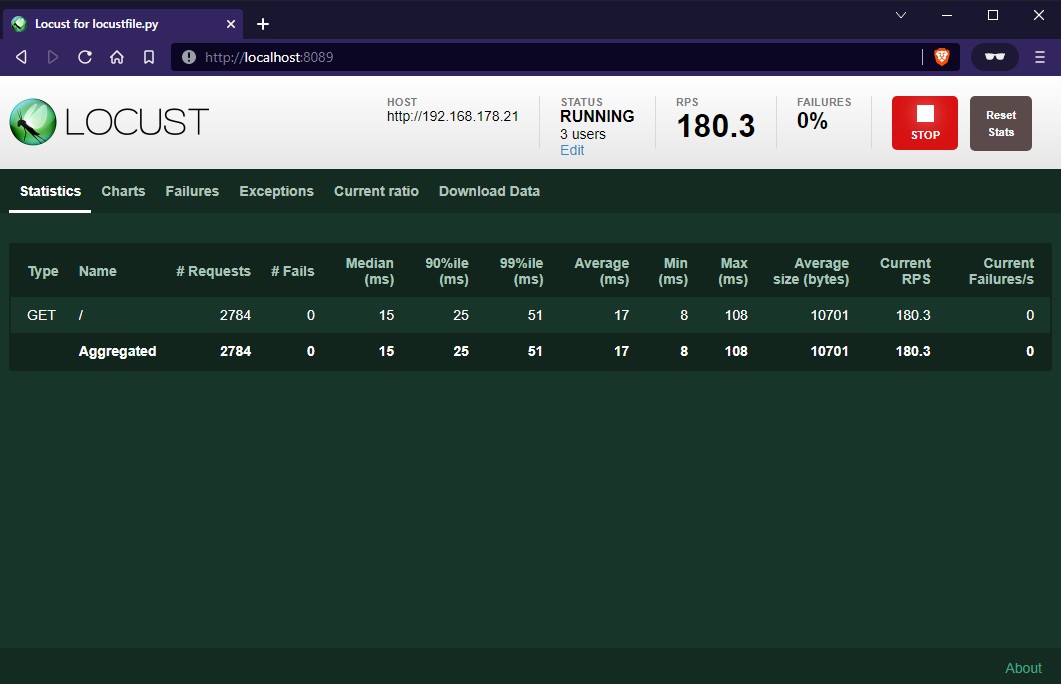
One you stop testing, you can analise your results using the different views that locust offers:
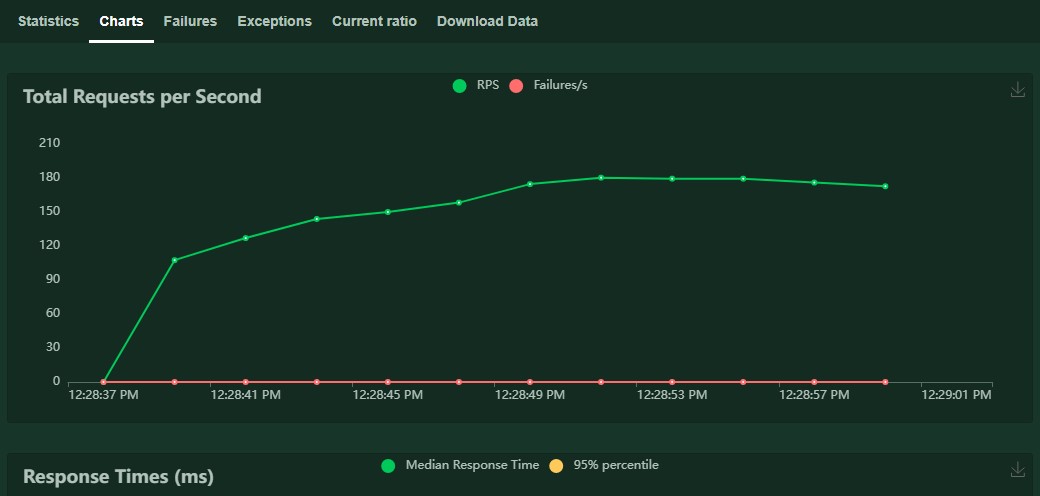
or you can simply preview the terminal view

Welldone! You have load tested a server using locust, congrats! 🎉
One step further, a more realistic test
Make a more realistic and suitable test by modifying the locustfile
to your needs
import time
from json import JSONDecodeError
from locust import HttpUser, task, between
class QuickstartUser(HttpUser):
# Waiting time after between each task ran by users
wait_time = between(1, 5)
@task
def hello_world(self):
self.client.get("/hello")
self.client.get("/world")
# "3" refers to the task's weight (it's 3 times more likely to be ran)
@task(3)
def view_items(self):
for item_id in range(10):
# group requests with different parameters together
self.client.get(f"/item?id={item_id}", name="/item")
time.sleep(1)
# call an API and validate its response
with self.client.post("/", json={"foo": 42, "bar": 69}, catch_response=True) as response:
try:
if response.json()["greeting"] != "hello":
response.failure("Did not get expected value in greeting")
except JSONDecodeError:
response.failure("Response could not be decoded as JSON")
except KeyError:
response.failure("Response did not contain expected key 'greeting'")
With locust you can test your server fairly quickly with its simple setup.
Its configuration is also well docummented, take a look at their official site: Locust documentation
Happy load testing! 🛩️
Load testing a server
2022 Jun 12 See all postsWhat is load testing? ⁉️
Quoting wikipedia:
Let's get started then! 🏁
Requirements
We are gonna load test our server using Locust, a simple and straight forward python library.
Install locust by running:
Test the installation:
Load testing
Create a python file
locustfile.py
and add the following code in it:In the same folder, start testing by running:
You should see something similar to:
Now, you can access http://localhost:8089
You will now see Locust UI
Testing
You will be able to see the stats of your ongoing test:
One you stop testing, you can analise your results using the different views that locust offers:
or you can simply preview the terminal view
One step further, a more realistic test
Make a more realistic and suitable test by modifying the
locustfile
to your needsWith locust you can test your server fairly quickly with its simple setup.
Its configuration is also well docummented, take a look at their official site: Locust documentation
Daniel Ratmiroff © - site made with: blogmaker - credit to Vitalik Buterin